Are you looking for the best PHP guide for beginners to kickstart your journey in web development? Whether you’re a complete newbie or someone looking to refine your skills, PHP is an essential programming language for creating dynamic and interactive web applications.
In this article, we’ll cover:
By the end of this guide, you’ll be equipped to code like a pro and create your own web applications using PHP.
PHP Guide for Beginners: What is PHP?
PHP (Hypertext Preprocessor) is a server-side scripting language used to develop web applications. It is widely known for its ease of use, compatibility with multiple databases, and its ability to handle dynamic web content. Learn more about Why PHP is Important for Web Development.
π PHP Guide for Beginners: Why You Should Learn PHP for Web Development?
PHP is a server-side scripting language that makes web pages dynamic and interactive. Hereβs why learning PHP is a great choice:
β
Easy to Learn β Simple syntax, beginner-friendly
β
Open-Source & Free β No cost to get started
β
Supports Databases β Works with MySQL, PostgreSQL, and more
β
Fast Performance β Executes code quickly on the server
β
Huge Community Support β Tons of resources, forums, and tutorials
Did you know? PHP is the backbone of platforms like WordPress, Joomla, and Laravel, making it an essential skill for web developers.
Setting Up Your PHP Development Environment
Before writing your first PHP program, you need a local development environment to run and test PHP scripts. Follow these steps:
1. Install a Local Server
To execute PHP scripts, you need a server environment. You can install:
πΉ XAMPP (Windows, Mac, Linux) β Download Here
πΉ WAMP (Windows) β Download Here
πΉ MAMP (Mac) β Download Here
2. Choose a Code Editor
You can write PHP code using:
πΉ VS Code β Lightweight and feature-rich
πΉ Sublime Text β Fast and efficient
πΉ PHPStorm β Best for professional PHP development
3. Run Your First PHP Script
Once installed, create a new file in your local serverβs htdocs
folder and name it index.php
. Add the following code:
<?php
echo "Hello, World! Welcome to PHP.";
?>
Save the file and run it in your browser by visiting:
π http://localhost/index.php
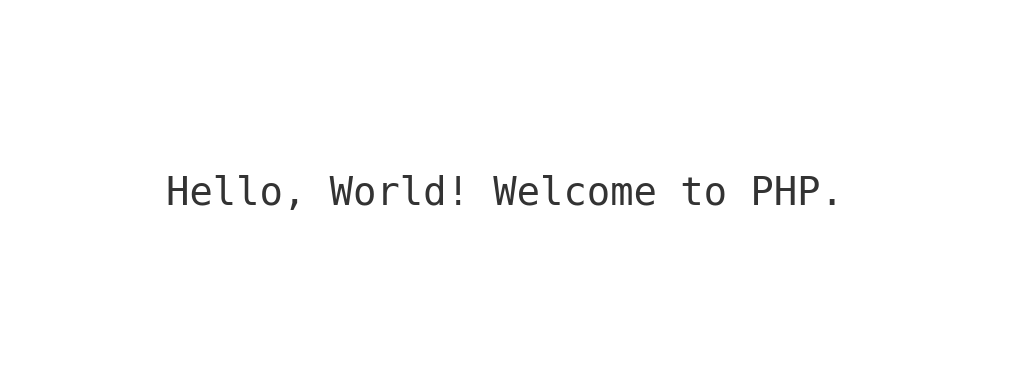
π Congratulations! You’ve just executed your first PHP script.
π Step-by-Step Guide to Learning PHP
1οΈβ£ Install PHP and Set Up Your Environment
Before coding, you need to set up a local development environment:
πΉ Download and Install XAMPP (Download Here)
πΉ Install VS Code or PHPStorm for writing PHP scripts
πΉ Test PHP by running:
<?php
echo "Hello, World!";
?>
πΉ Open localhost
in your browser to see the output
2οΈβ£ Understanding PHP Basics
PHP syntax is easy! Here are the key concepts:
β Variables
$name = "John";
echo "Hello, $name";
β PHP Arrays
$colors = array("Red", "Blue", "Green");
echo $colors[0]; // Output: Red
β Functions
function greet() {
return "Welcome to PHP!";
}
echo greet();
β Conditional Statements
$age = 18;
if ($age >= 18) {
echo "You can vote!";
} else {
echo "You are too young.";
}
β Loops
for ($i = 1; $i <= 5; $i++) {
echo "Number: $i <br>";
}
3οΈβ£ Work with Forms and User Input
PHP is great for handling form submissions:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = $_POST['name'];
echo "Hello, $name!";
}
?>
<form method="post">
<input type="text" name="name" placeholder="Enter your name">
<button type="submit">Submit</button>
</form>
4οΈβ£ Connect PHP with MySQL Database
PHP works well with databases to store and retrieve data:
$conn = mysqli_connect("localhost", "root", "", "mydb");
if ($conn) {
echo "Connected successfully!";
}
π Learn more about PHP & MySQL: PHP MySQL Guide
5οΈβ£ Build Your First Web Project
Want to create your own web project? Try building:
β A simple contact form
β A user login system
β A blog using PHP & MySQL
π Best Practices for PHP Development
β Always sanitize user input to prevent SQL injection
β Use prepared statements when working with databases
β Follow MVC architecture for cleaner code
β Keep PHP and third-party libraries updated
β Debug with var_dump() and error_reporting()
π Frequently Asked Questions (FAQs)
1. Is PHP still worth learning in 2024?
Yes! PHP is widely used in web development, and platforms like WordPress, Shopify, and Laravel rely on it.
2. Can I learn PHP in a month?
Yes! If you dedicate 1-2 hours daily, you can learn PHP basics and build a simple project in 30 days.
3. Do I need to learn MySQL with PHP?
Itβs recommended. PHP & MySQL together allow you to store and manage data for web applications.
4. Which is better: PHP or Python for web development?
Both are great! PHP is best for traditional web applications, while Python (Django, Flask) is better for AI & data science.
5. Where can I practice PHP online?
You can use PHP Sandbox (PHP Fiddle) or install XAMPP to practice locally.
π Conclusion
This PHP guide for beginners covers everything you need to start coding like a pro. From setting up your development environment to building your first PHP project, you now have the skills to begin your PHP journey.
π‘ Start coding today and bring your web projects to life with PHP! π